Published
- 2 min read
Postman pre-request script that captures tokens
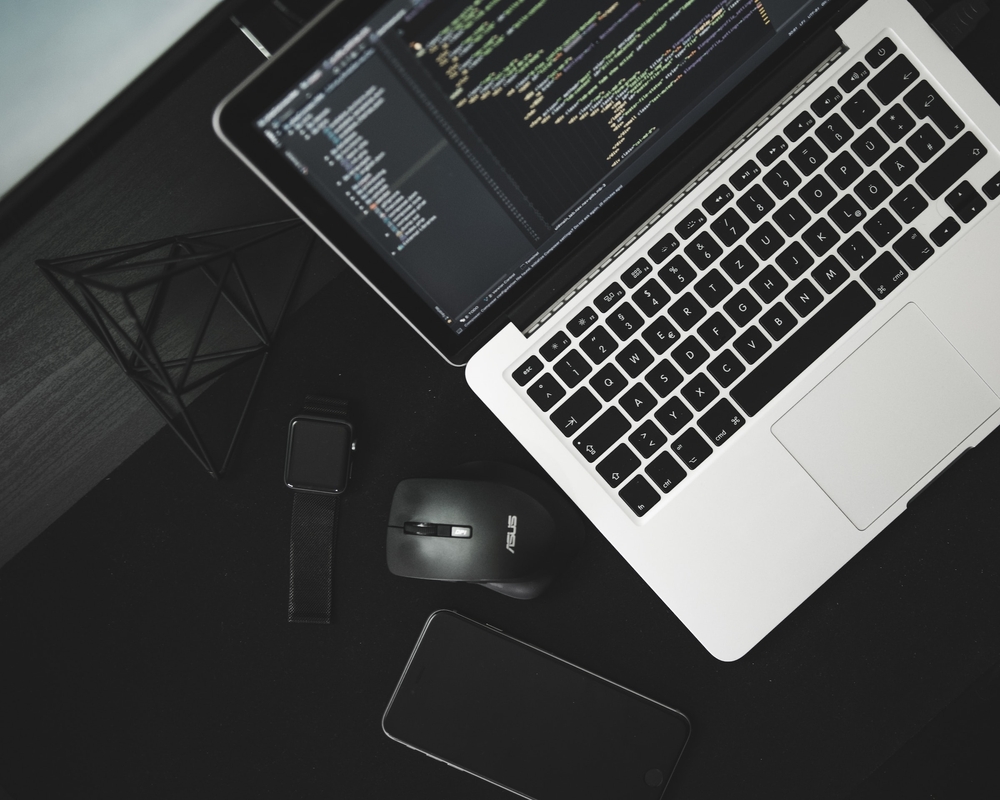
Creating a Postman pre-script to set tokens
This post details dives into devloping a pre-request script in Postman that obtains an access token from the authentication server using the client credentials grant
1. Define ID’s and secrets
First we define required credentials to generate an access token.
// Set your credentials
var clientId = 'xxxxxxxxxxxxxxxxxxxxxxxxx'
var clientSecret = 'xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'
2. Build request URL and parameters
Next we build out the request URL, headers and request body
// Set the URL and request parameters
var url = 'https://xxx-xxx.xxxxxxxx.com/oauth2/token'
var headers = {
'Content-Type': 'application/x-www-form-urlencoded',
Authorization: 'Basic ' + btoa(clientId + ':' + clientSecret)
}
// Set the request body
var body = {
mode: 'urlencoded',
urlencoded: [{ key: 'grant_type', value: 'client_credentials' }]
}
3. Send the post request with pm.sendRequest
Next we make a request using pm.sendRequest
function with our defined url
, headers
and body
// Send the POST request
pm.sendRequest({
url: url,
method: 'POST',
header: headers,
body: body,
secure: true
}, function (err, res) {
// Handle the error
if (err) {
console.error('Error:', err);
return;
}
4. Extract the accessToken and set with pm.environment.set
Using the returned response, we can now save and set the global variable accessToken
with postman’s pm.environment.set
function
// Extract and save accessToken as a variable
var jsonResponse = res.json()
if (jsonResponse && jsonResponse.access_token) {
pm.environment.set('accessToken', jsonResponse.access_token)
console.log('Access Token saved:', pm.environment.get('accessToken'))
}