Published
- 3 min read
Building Powerful Language AI Applications with Langchain
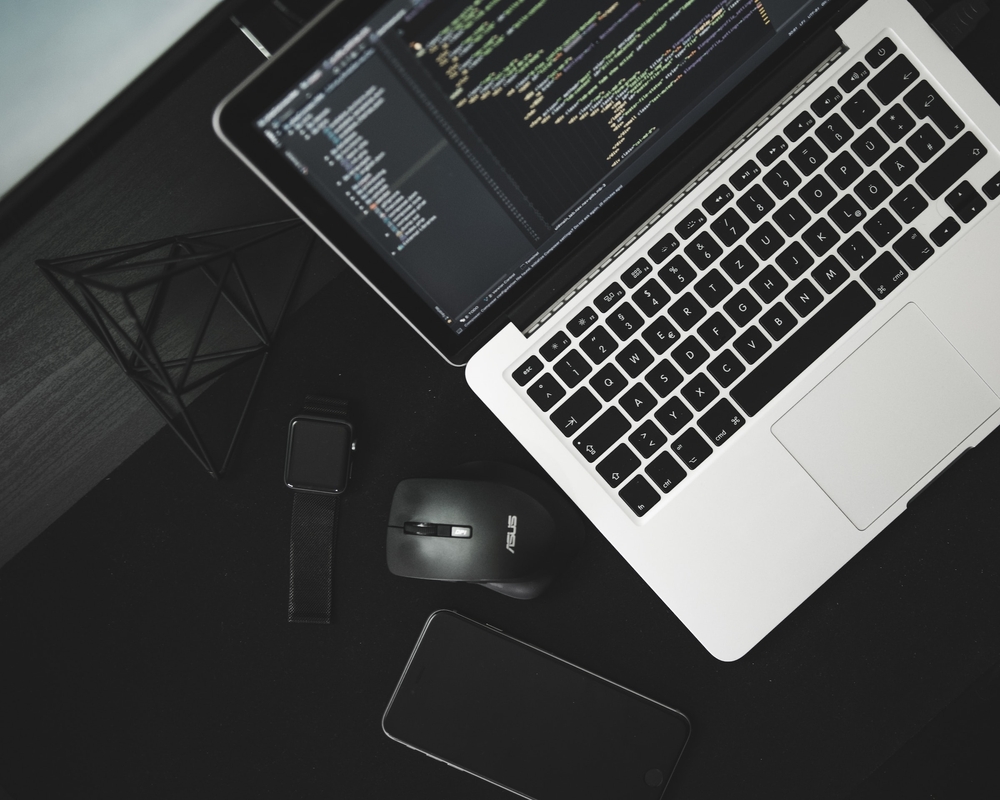
LangChain Setup: Building Powerful Language AI Applications
Ready to supercharge your AI development with LangChain? Dive in and start building sophisticated language AI systems for complex task automation! 🚀
Introduction to LangChain
LangChain is a powerful framework for developing applications powered by language models. It provides a comprehensive set of tools and components for building advanced AI systems that can understand, process, and generate human language.
Setting Up LangChain
Installation
To get started with LangChain, you’ll need to install it using pip:
pip install langchain
Basic Usage
Here’s a simple example of how to create a chain using LangChain:
from langchain import PromptTemplate, LLMChain
from langchain.llms import OpenAI
# Initialize the language model
llm = OpenAI(temperature=0.9)
# Define a prompt template
prompt = PromptTemplate(
input_variables=["product"],
template="What is a good name for a company that makes {product}?",
)
# Create the chain
chain = LLMChain(llm=llm, prompt=prompt)
# Run the chain
print(chain.run("eco-friendly water bottles"))
Key Features of LangChain
-
Modular Components: LangChain offers various components for working with language models, including prompts, memory, and indexes.
-
Off-the-shelf Chains: Pre-assembled component chains for specific tasks, making it easy to get started quickly.
-
Flexible Integration: Supports multiple language models and can be easily integrated with other tools and APIs.
-
Memory Management: Built-in support for managing conversation history and context.
-
Data Awareness: Ability to connect language models with external data sources for more informed responses.
Advanced Usage
Using Agents
LangChain allows you to create agents that can use tools to accomplish tasks:
from langchain.agents import load_tools
from langchain.agents import initialize_agent
from langchain.agents import AgentType
from langchain.llms import OpenAI
# Initialize the language model
llm = OpenAI(temperature=0)
# Load some tools to use
tools = load_tools(["serpapi", "llm-math"], llm=llm)
# Create an agent
agent = initialize_agent(tools, llm, agent=AgentType.ZERO_SHOT_REACT_DESCRIPTION, verbose=True)
# Run the agent
agent.run("What was the high temperature in SF yesterday in Fahrenheit? What is that number raised to the .023 power?")
Security Considerations
When using LangChain, it’s important to be aware of potential security vulnerabilities. A critical security flaw (CVE-2023-36258) was recently discovered that could allow arbitrary code execution 1. To mitigate this risk:
- Always validate and sanitize input to remove potentially harmful code.
- Implement command whitelisting to only allow approved commands.
- Consider using user consent mechanisms before executing dynamically generated code.
Conclusion
LangChain is a versatile and powerful framework for building language AI applications. With its modular design and extensive features, it offers developers the tools to create sophisticated AI systems capable of handling complex language tasks.
As you explore LangChain, remember to keep an eye on the official documentation, which has recently undergone a significant refresh for version 0.2 2. The new documentation structure includes tutorials, how-to guides, conceptual guides, and API reference, making it easier to find the information you need.
Give LangChain a try and unlock the potential of language models in your AI projects!